Stable Diffusion Scripts
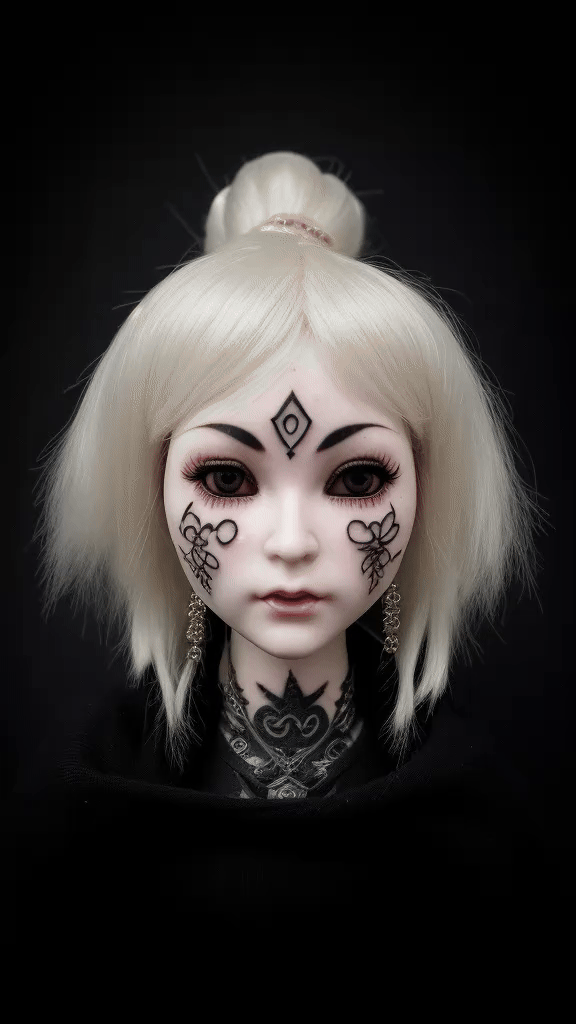
Overview
Data Grooming
Numbering PNG files in a folder in sequence
1import os
2import pathlib
3
4try:
5 collection = os.getcwd()
6 num_files_renamed = 0
7 for i, filename in enumerate(os.listdir(collection)):
8 file_extension = pathlib.Path(filename).suffix
9 if file_extension == ".png" or file_extension == ".jpg":
10 new_filename = f"{str(i).zfill(5)}.png"
11 old_path = os.path.join(collection, filename)
12 new_path = os.path.join(collection, new_filename)
13 os.rename(old_path, new_path)
14 num_files_renamed += 1
15 print(f"Renamed file {filename} to {new_filename}")
16 print(f"Renamed {num_files_renamed} files.")
17except Exception as e:
18 print(f"Error occurred: {e}")
This Python script renames all PNG and JPG files in the current working directory by adding a sequential number to the beginning of the filename, padded with leading zeros, and changing the file extension to PNG. It uses the os and pathlib modules to access the file system and the try-except block to catch any errors that may occur during file renaming. The script also prints progress messages to the console, showing the original and new filenames of each file that is renamed.
Delete every other image in a folder
1import os
2
3# get the current working directory
4cwd = os.getcwd()
5
6# get a list of all the files in the directory
7files = os.listdir(cwd)
8
9# loop through the list of files
10for i, file in enumerate(files):
11 # check if the file is an image file
12 if file.endswith('.jpg') or file.endswith('.jpeg') or file.endswith('.png'):
13 # delete every other image file
14 if i % 2 == 1:
15 os.remove(file)
Distance Sort
1import os
2from PIL import Image
3from math import sqrt
4
5folder_path = 'C:/Users/trima/FILM/photos'
6image_files = os.listdir(folder_path)
7
8# Open the first image and get its RGB values
9first_image = Image.open(os.path.join(folder_path, image_files[0]))
10first_image_rgb = first_image.getdata()
11
12# Create a list to store the distances
13distances = []
14
15# Iterate over the remaining images in the folder
16for image_file in image_files[1:]:
17 if image_file.endswith('.png'):
18 image = Image.open(os.path.join(folder_path, image_file))
19 image_rgb = image.getdata()
20 distance = 0
21 for i in range(len(first_image_rgb)):
22 distance += sqrt((first_image_rgb[i][0]-image_rgb[i][0])**2 + (first_image_rgb[i][1]-image_rgb[i][1])**2 + (first_image_rgb[i][2]-image_rgb[i][2])**2)
23 print((distance, image_file))
24 distances.append((distance, image_file))
25
26# Sort the distances list by distance
27distances.sort()
28
29# Rename the first image to "0000.png"
30os.rename(os.path.join(folder_path, image_files[0]), os.path.join(folder_path, "0000.png"))
31
32# Rename each image to the next number in the sequence
33for i in range(len(distances)):
34 os.rename(os.path.join(folder_path, distances[i][1]), os.path.join(folder_path, str(i+1).zfill(4) + '.png'))
This script is a Python script that renames a sequence of PNG images in a folder based on the distance of their RGB values from the RGB values of the first image in the sequence. The script imports the os, PIL and math modules, it sets the folder path where the images are located and get the list of all files in that folder. It opens the first image and gets its RGB values, then creates an empty list to store the distances. It iterates over the remaining images in the folder, if the file is a png it opens the image and gets its RGB values. Then it calculates the distance between the RGB values of the current image and the first image using the Euclidean distance formula, appends the distance and the image name to the distance list. The script then sorts the distance list by the distance, renames the first image to "0000.png" and renames each image to the next number in the sequence using the os.rename() function, and 4 digits zero-padded number followed by the extension.
Color Grading
By Mean
1import os
2import cv2
3import numpy as np
4
5def average_color_grading():
6 # Get all image filenames in the same directory as the script
7 filenames = [f for f in os.listdir() if f.endswith('.jpg') or f.endswith('.png')]
8
9 # Initialize a sum of color grading for all images
10 color_grading_sum = None
11
12 # Iterate through all images, adding each image's color grading to the sum
13 for filename in filenames:
14 print("averaging " + filename)
15 image_path = filename
16 image = cv2.imread(image_path)
17
18 # Average color grading of an image is computed as mean of its pixels
19 color_grading = np.mean(image, axis=(0, 1))
20
21 # Add the color grading of the current image to the sum
22 if color_grading_sum is None:
23 color_grading_sum = color_grading
24 else:
25 color_grading_sum += color_grading
26
27 # Divide the sum of color grading by the number of images to get the average color grading
28 average_color_grading = color_grading_sum / len(filenames)
29
30 return average_color_grading
31
32def apply_color_grading(average_color_grading):
33 # Get all image filenames in the same directory as the script
34 filenames = [f for f in os.listdir() if f.endswith('.jpg') or f.endswith('.png')]
35
36 # Create a new folder to save the color graded frames
37 color_graded_folder = os.path.join(os.getcwd(), 'color_graded')
38 os.makedirs(color_graded_folder, exist_ok=True)
39
40 # Iterate through all images, applying the average color grading to each frame
41 for i, filename in enumerate(filenames):
42 print("color grading " + filename)
43 image_path = filename
44 image = cv2.imread(image_path)
45
46 # Subtract the average color grading from each pixel to apply the color grading
47 color_graded_image = image - np.mean(image, axis=(0, 1)) + average_color_grading
48
49 # Zero-pad the sequential number and save the color graded image with the zero-padded sequential number
50 color_graded_image_path = os.path.join(color_graded_folder, str(i).zfill(5) + '.png')
51 cv2.imwrite(color_graded_image_path, color_graded_image)
52
53average_color_grading = average_color_grading()
54apply_color_grading(average_color_grading)
This program applies color grading to a set of images stored in the "images" folder. It does so by first computing the average color grading of all the images and then subtracting the average color grading from each pixel of each image and adding the average color grading. The resulting color graded images are saved in a new folder called "color_graded" within the "images" folder. It applies the average color grading to each frame by subtracting the mean of each frame's pixels from each pixel and adding the average color grading.
Generate CFG values for X/Y plot
1let frames = 60;
2let str = "";
3function setup() {
4 noLoop();
5 for (let i = 0; i <= frames; i++) {
6 let x = map(i, 0, frames, 6, 9);
7 str += nf(x,1,2);
8 str += i < frames ? ", " : "";
9 }
10 print(str);
11}
Add a vignette fade
Fades from bottom to top. Great for hiding mistakes and artifacts.
1from PIL import Image
2import os
3import threading
4
5# Get the directory where the script is located
6script_dir = os.path.dirname(os.path.abspath(__file__))
7
8class VignetteThread(threading.Thread):
9 def __init__(self, filename):
10 threading.Thread.__init__(self)
11 self.filename = filename
12
13 def run(self):
14 # Open the image file
15 image = Image.open(os.path.join(script_dir, self.filename))
16
17 # Define the size of the vignette black fade
18 fade_height = 256
19
20 # Create a black mask with the same size as the image
21 mask = Image.new("L", image.size, 255)
22
23 # Draw a linear gradient from white to black on the mask
24 for y in range(image.size[1] - fade_height, image.size[1]):
25 alpha = int(255 * (y - (image.size[1] - fade_height)) / fade_height)
26 mask.paste(255 - alpha, (0, y, image.size[0], y+1))
27
28 # Apply the mask to the image
29 image.putalpha(mask)
30
31 # Save the modified image with a new filename
32 new_filename = os.path.splitext(self.filename)[0] + "_vignette.png"
33 image.save(os.path.join(script_dir, new_filename))
34
35# Loop through each file in the directory and create a thread for each image
36threads = []
37for filename in os.listdir(script_dir):
38 if filename.endswith(".jpg") or filename.endswith(".png"):
39 thread = VignetteThread(filename)
40 threads.append(thread)
41 thread.start()
42
43# Wait for all threads to complete
44for thread in threads:
45 thread.join()
Pixelate
Stable Diffusion is pretty bad at making convincing looking pixel art. This script post-processes images by average 4x4 clusters of pixels as well as rounding RGB values to MOD4 to reduce the color palette.
1from PIL import Image
2import os
3
4# Define the pixel size of the grid
5GRID_SIZE = 4
6
7# Helper function to round a color channel to the nearest multiple of 4
8def round_to_mod4(value):
9 return 4 * round(value / 4)
10
11# Create the output directory if it doesn't exist
12if not os.path.exists('output'):
13 os.makedirs('output')
14
15# Iterate through all files in the current directory
16for filename in os.listdir('.'):
17 if filename.endswith('.png') or filename.endswith('.jpg'):
18 # Open the image and get its size
19 image = Image.open(filename)
20 width, height = image.size
21
22 # Create a new blank image to hold the pixelated version
23 pixelated = Image.new('RGB', (width, height), color='white')
24
25 # Loop through each 2x2 pixel grid in the image
26 for x in range(0, width, GRID_SIZE):
27 for y in range(0, height, GRID_SIZE):
28 # Get the colors of the 4 pixels in the grid
29 colors = []
30 for i in range(GRID_SIZE):
31 for j in range(GRID_SIZE):
32 if x+i < width and y+j < height:
33 colors.append(image.getpixel((x+i, y+j)))
34
35 # Calculate the average color of the grid, rounding each channel to the nearest multiple of 4
36 avg_color = tuple(round_to_mod4(sum(c)/len(c)) for c in zip(*colors))
37
38 # Set all 4 pixels in the grid to the average color
39 for i in range(GRID_SIZE):
40 for j in range(GRID_SIZE):
41 if x+i < width and y+j < height:
42 pixelated.putpixel((x+i, y+j), avg_color)
43
44 # Save the pixelated image with a new filename in the output directory
45 new_filename = 'pixelated_' + filename
46 output_path = os.path.join('output', new_filename)
47 pixelated.save(output_path)